Building And Releasing Your Capacitor Android App
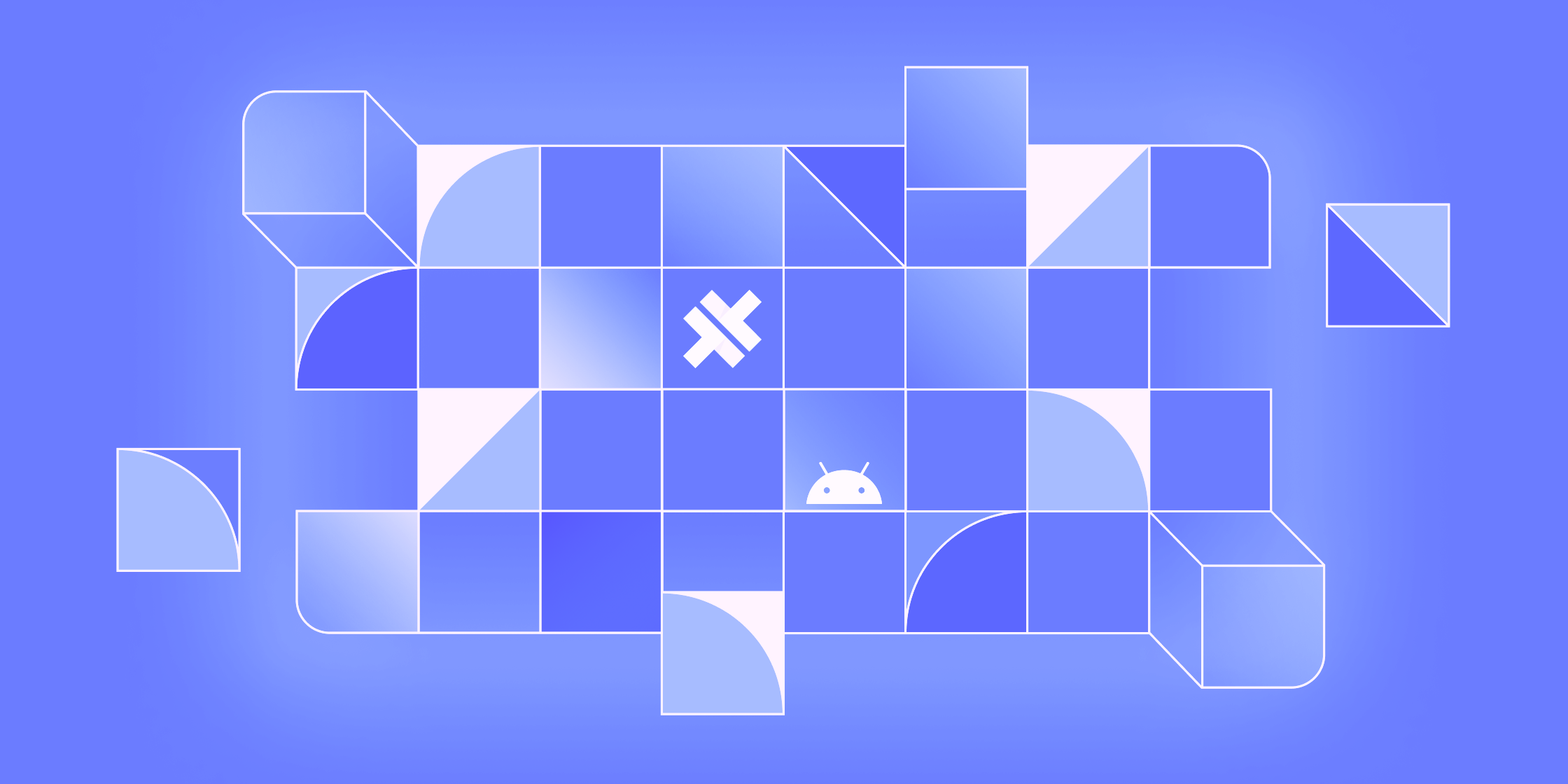
This is a guest post from Simon Grimm, Ionic Developer Expert and educator at the Ionic Academy, an online school with 70+ video courses focused entirely on building awesome mobile apps with Ionic!
You just built your first Ionic App and want to show it to the world, but you have no idea how to submit it to the Store? Then you have come to the right place.
In this guide, you will learn all the steps required to go from an Ionic app to a released Android app. You don’t need to have any previous experience with building native mobile apps to follow along. All you need is basic knowledge of how to build modern web applications!
Prerequisite
What is Capacitor?
Capacitor is a native runtime that gives modern web application access to native APIs such as Camera, File System, Notifications, Network, GPS, and more using JavaScript. In a nutshell, Ionic is for building UIs, and Capacitor makes it possible to build and run your app on a native device. It comes with a very slim API, so a lot of the build and deploy process we cover is actually just using the native iOS and Android tools!
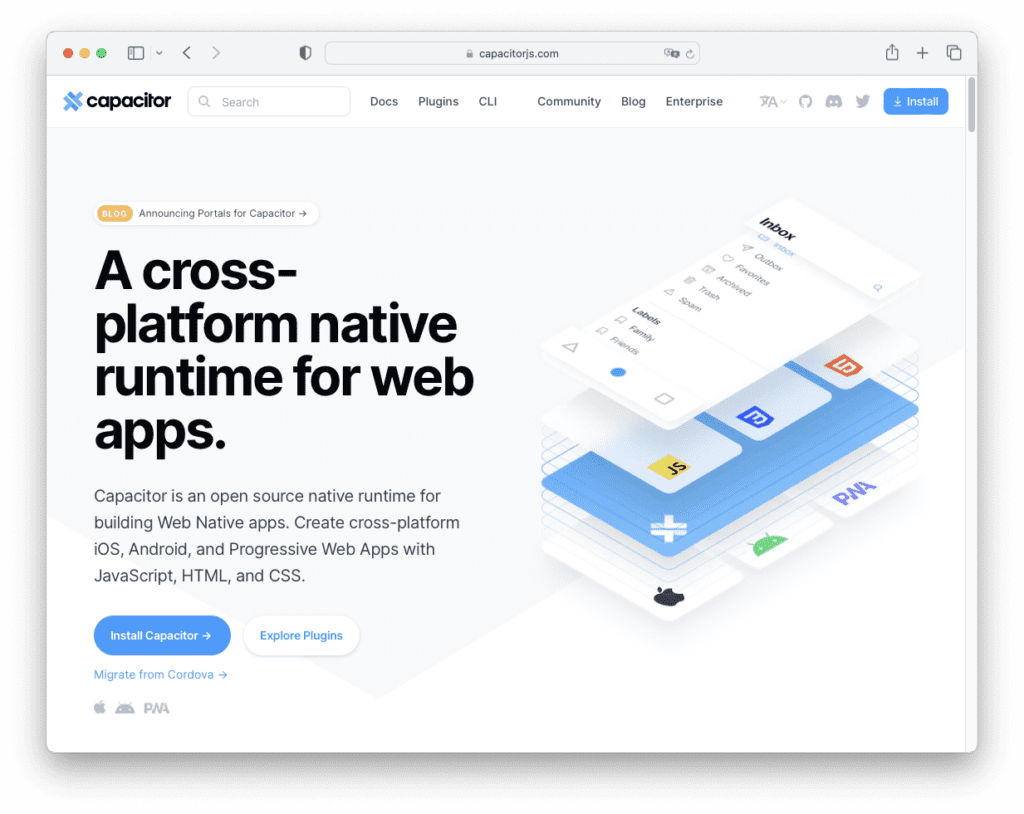
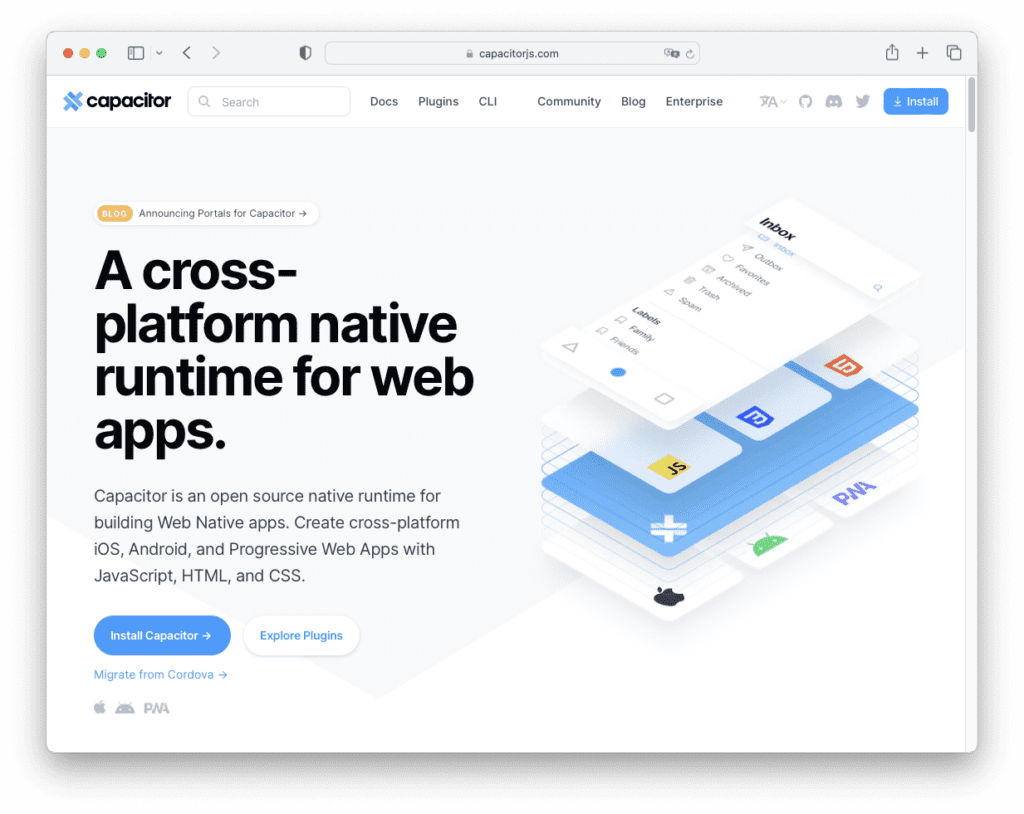
Install Native Tools
To later build our app, we rely on the tools that native developers use as well.
This means installing Android Studio to download and manage your Android SDK.
Once you reach the last step of submitting an app, you also need to be enrolled in the Google developer program, which is a one-time fee of $25.
App Preparation
For simplicity, I will explain all steps based on a sample Ionic React app. Most likely you arrived at this guide with an app yourself, so let’s get started with the configuration of our project!
Your Capacitor Configuration
Your project already comes with a capacitor.config.ts in which you can define some general settings.
First, you should make sure that you replace the default appId with an actual bundle ID that matches your company/app name. This is a unique identifier that we will later use for Android, so change it to something specific to your company or project.
Adding Native Platforms with Capacitor
To build your Ionic app with Capacitor, we need to create a native project for Android.
Make sure you also run the build command before, because Capacitor will sync your web build to the native platform folder – which is essentially the magic of Capacitor.
Later on, you will simply update your code and sync the changes again, for now, get started by running:
# Build your app and add the Android platform
ionic build
ionic cap add android
At this point, you can open your native projects and deploy the app to a device or simulator:
ionic cap open android
Alright, let’s continue with another important piece of the deployment puzzle.
Generating Splashscreen and App Icon
Every app needs a cool icon and splash screen, which is the first screen users see when starting your app later. For this task, we can use the Capacitor assets plugin, and to get started you should add an icon to a new assets folder at the root of your project:
assets/
├── icon.png
└── splash.png
Make sure you are using the right dimensions for those files:
• assets/icon.(png|jpg) must be at least 1024×1024px
• assets/splash.(png|jpg) must be at least 2732×2732px
Now we can install the Capacitor assets plugin and run the generate command in our project:
npm install @capacitor/assets
npx capacitor-assets generate
This will generate the icon and splash in different dimensions, and if you get a success message, you can see that the images were updated in your native projects:
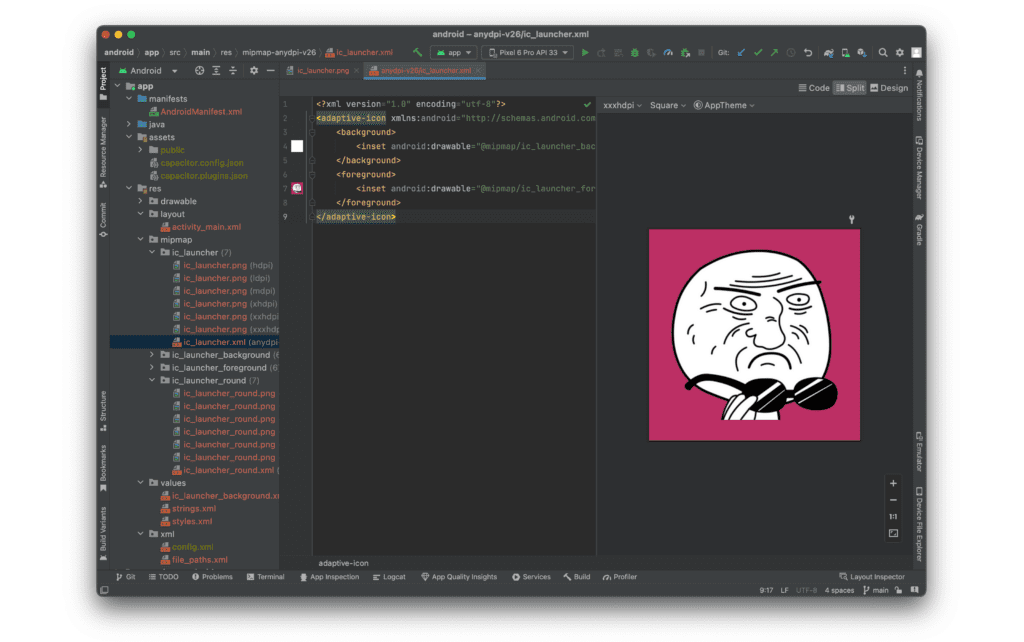
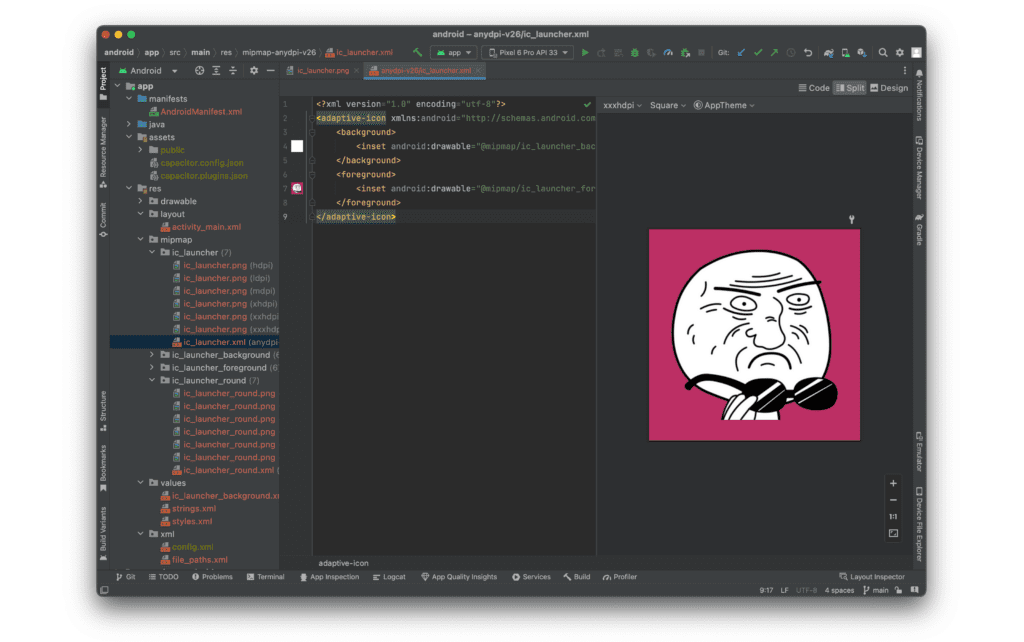
Now let’s get that app released!
Android Play Store Deployment
To publish your app on the Play Store, you need to sign your native app with a digital signature. In case you missed it, you also need a Google Play Developer account to upload and submit your app.
Generating a Signed Bundle with Android Studio
There are actually multiple ways to build your Android app, but let’s start with the easiest.
First, let’s build our app from the command line to make sure we have the latest web assets in your native project:
ionic cap build android --prod
ionic cap open android
Now, we can select the Build menu and click on Generate Signed Bundle/APK
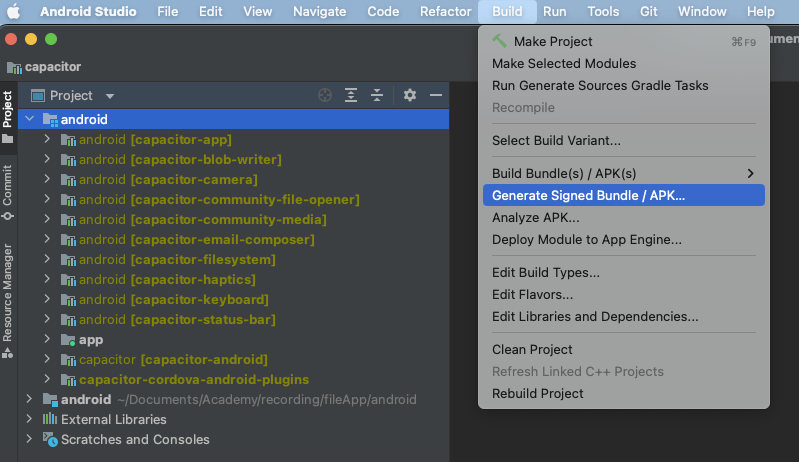
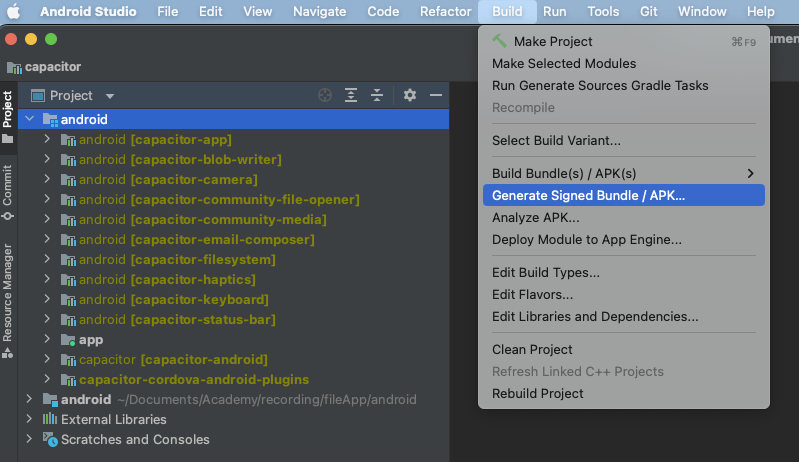
This will open a new window in which we select Android App Bundle. Click next, and you will get a dialog to choose or create a key store.
The key store is the way you can verify with Android/Google that you are the owner/developer of the app. If this is your first time going through this (or it’s a new app) you can simply click on Create new… to create a new key store file.
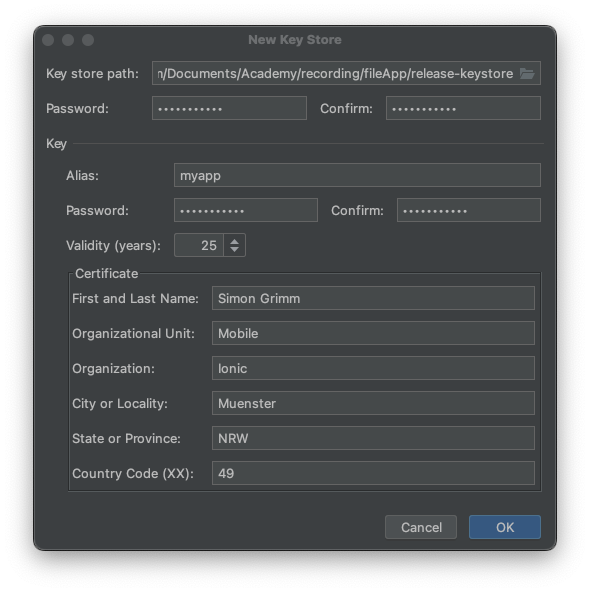
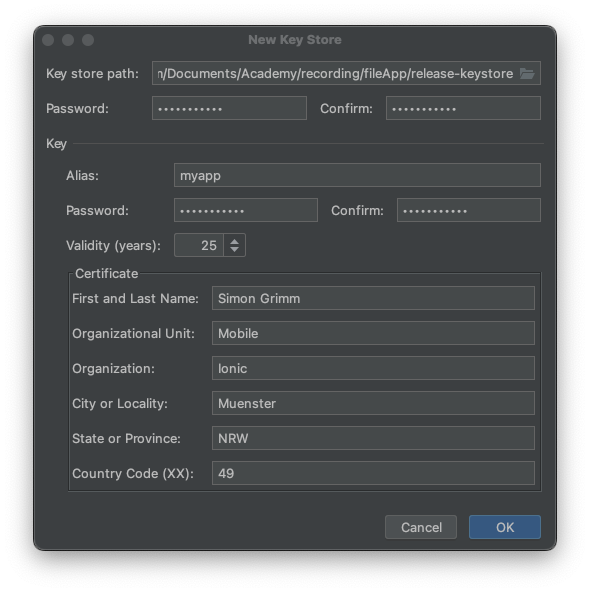
After filling in the data and clicking Ok, that information should be automatically used in the last open dialog to create our signed bundle:
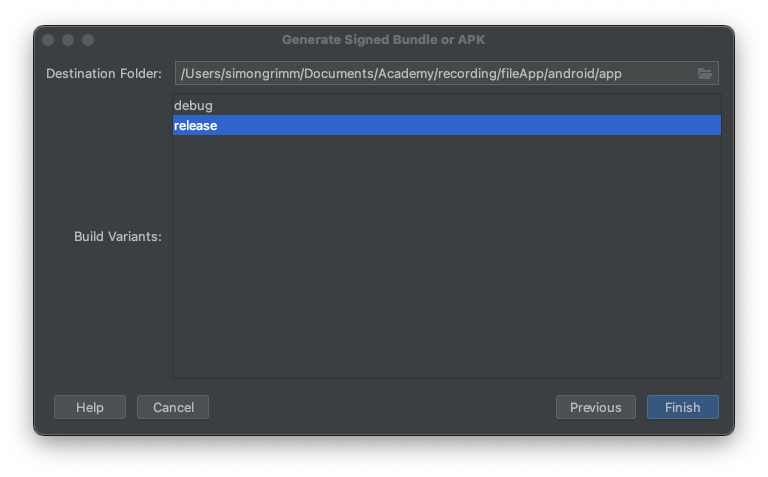
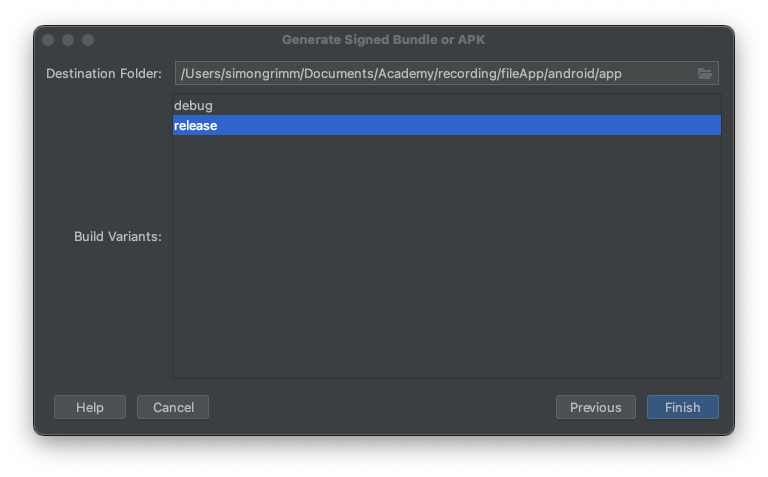
If the build is successful, you should pretty soon find a file under android/app/release/app-release.aab in your project.
Generating a Bundle through the Terminal
Alternatively, if you prefer to work through the CLI, or have a CI/CD pipeline, you can sign your app with the command line tools provided by Android. For this, we can now add a new file at android/keystore.properties and insert our key store credentials:
storePassword=yourstorepassword
keyPassword=yourkeypassword
keyAlias=yourkeyalias
storeFile=./../../release-keystore
Now we need to load this file inside the android/app/build.gradle, load the values into a signingConfigs
object, and add this to the release
build type like this:
apply plugin: 'com.android.application'
def keystorePropertiesFile = rootProject.file("keystore.properties")
def keystoreProperties = new Properties()
keystoreProperties.load(new FileInputStream(keystorePropertiesFile))
android {
compileSdkVersion rootProject.ext.compileSdkVersion
defaultConfig {
applicationId "com.devdactic.filesapp"
minSdkVersion rootProject.ext.minSdkVersion
targetSdkVersion rootProject.ext.targetSdkVersion
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
aaptOptions {
ignoreAssetsPattern '!.svn:!.git:!.ds_store:!*.scc:.*:!CVS:!thumbs.db:!picasa.ini:!*~'
}
}
signingConfigs {
release {
keyAlias keystoreProperties['keyAlias']
keyPassword keystoreProperties['keyPassword']
storeFile file(keystoreProperties['storeFile'])
storePassword keystoreProperties['storePassword']
}
}
buildTypes {
release {
signingConfig signingConfigs.release
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
As a result, you can now perform a build directly from your terminal by navigating into the android folder and directly calling the Gradle build tools to create a release bundle:
cd ./android
./gradlew bundleRelease
If the build is successful, you can find the resulting bundle at android/app/build/outputs/bundle/release/app-release.aab in your project.
Submitting your Android Build
Now, regardless of which approach you took, you should have an AAB file that can build uploaded to the Play Store. Let’s visit the Google Play Store Developer Console and create a new app.
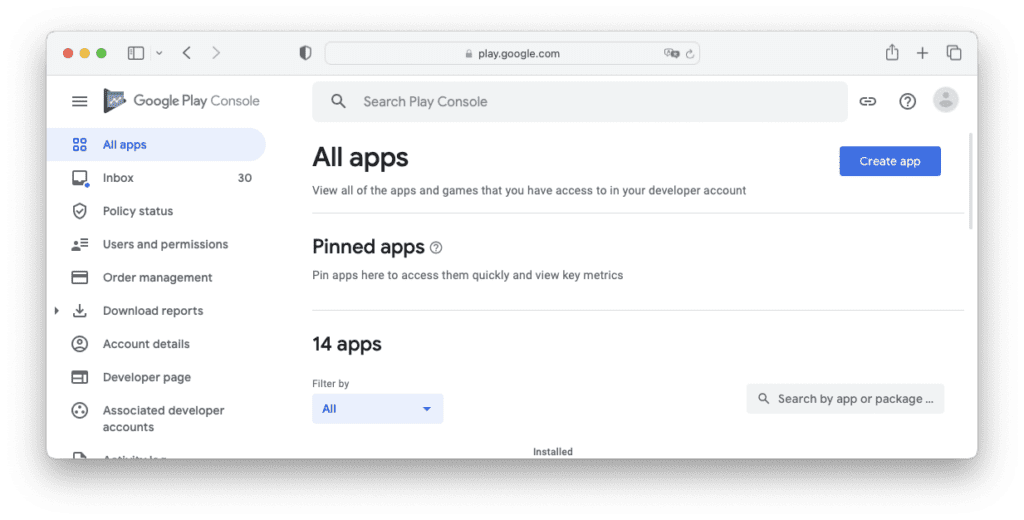
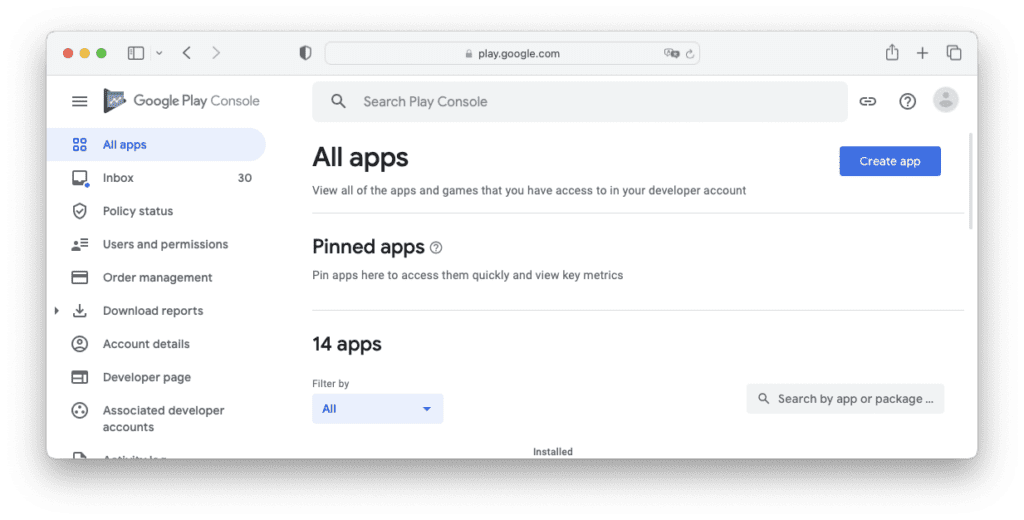
Fill out the form about general app information and click Create app to add a new app to your Play console!
You will now arrive at the dashboard of your project, from where you can scroll down to the Set-up your app section and expand all required tasks that you need to finish before releasing your app:
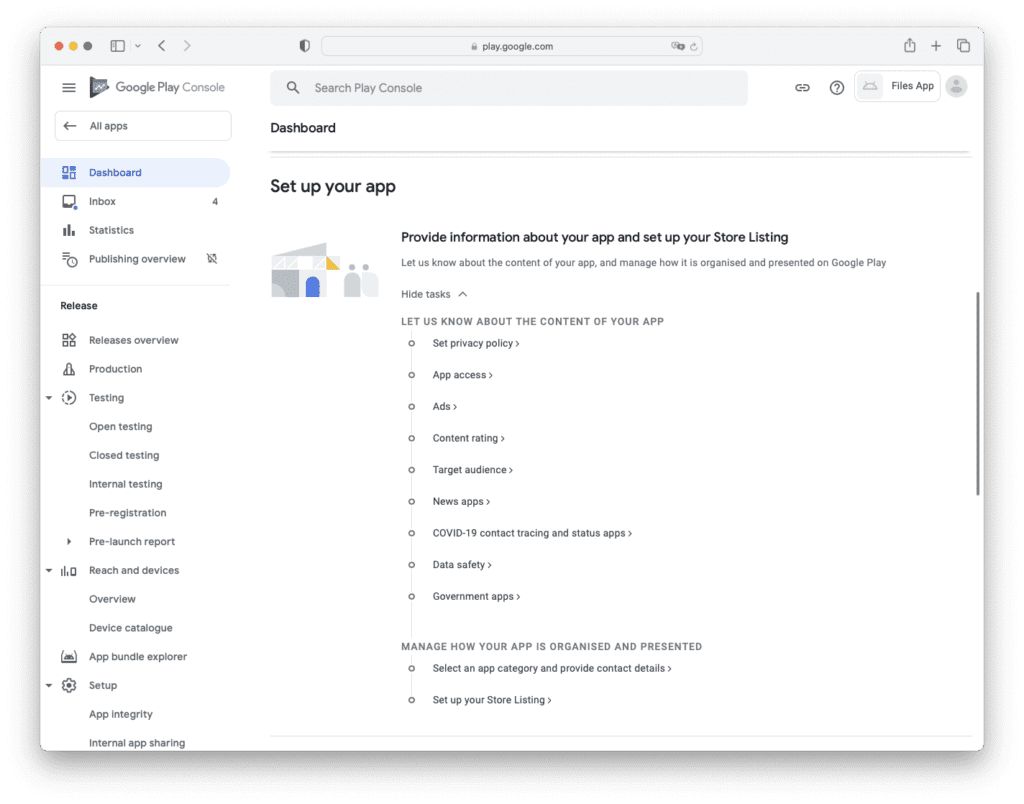
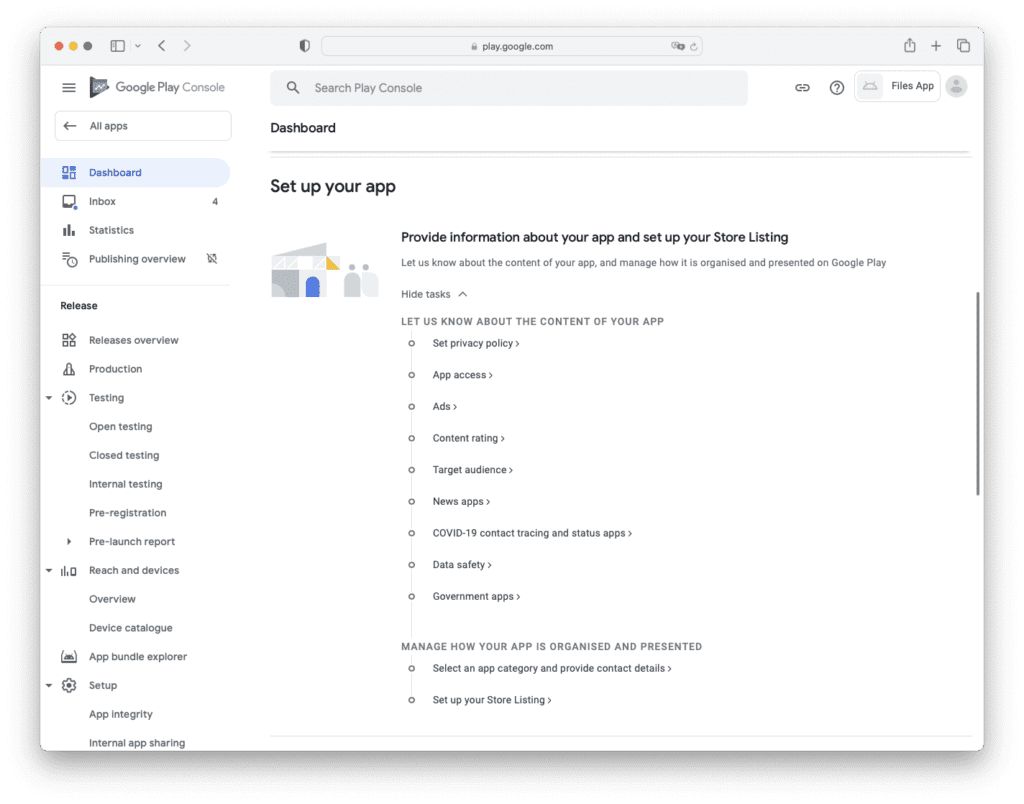
We will not go through all the forms, simply check all tasks and fill in the required information about your app!
After you are done you can head over to Production from where we click on Create new release.
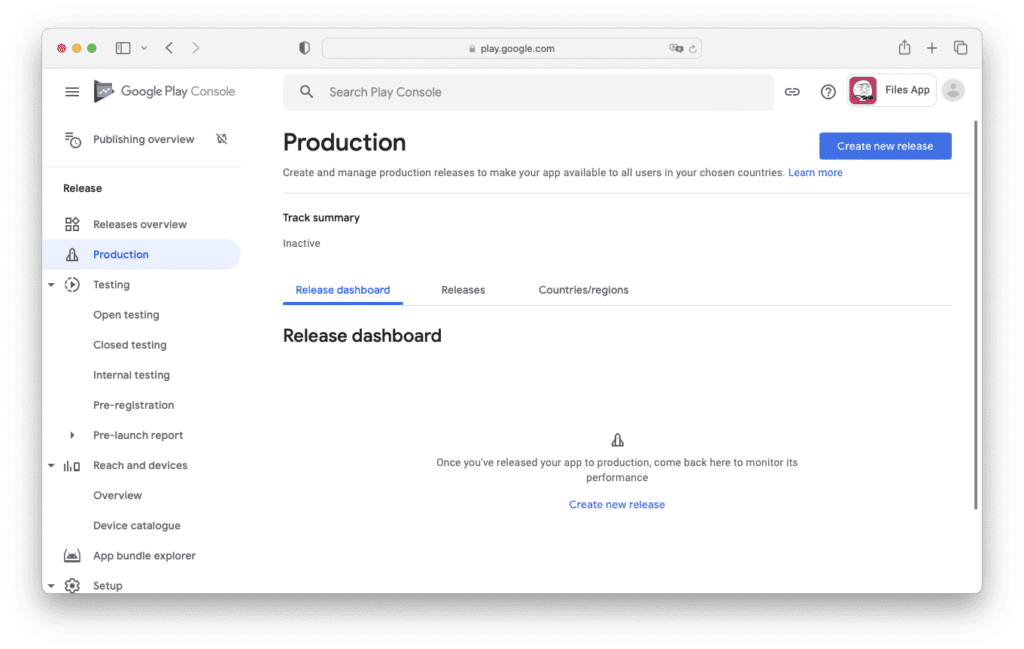
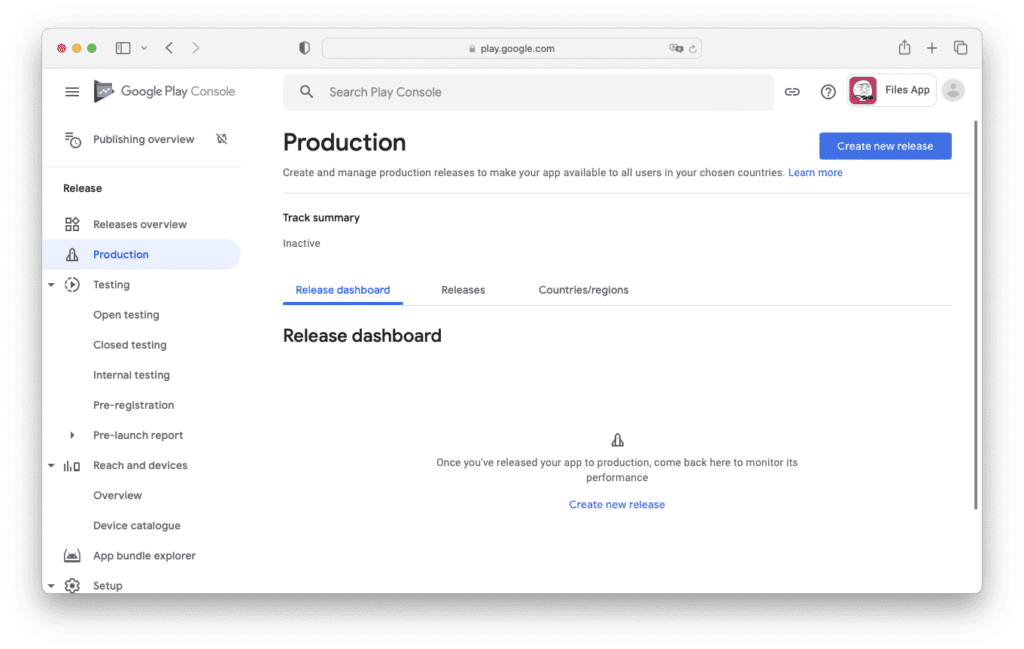
Note: We haven’t touched testing in here, but you could instead upload your build to the open/closed testing track of Google Play and start rolling out to specific testers before creating your production release!
Now the most important step happens as we drop our aab bundle into the upload area!
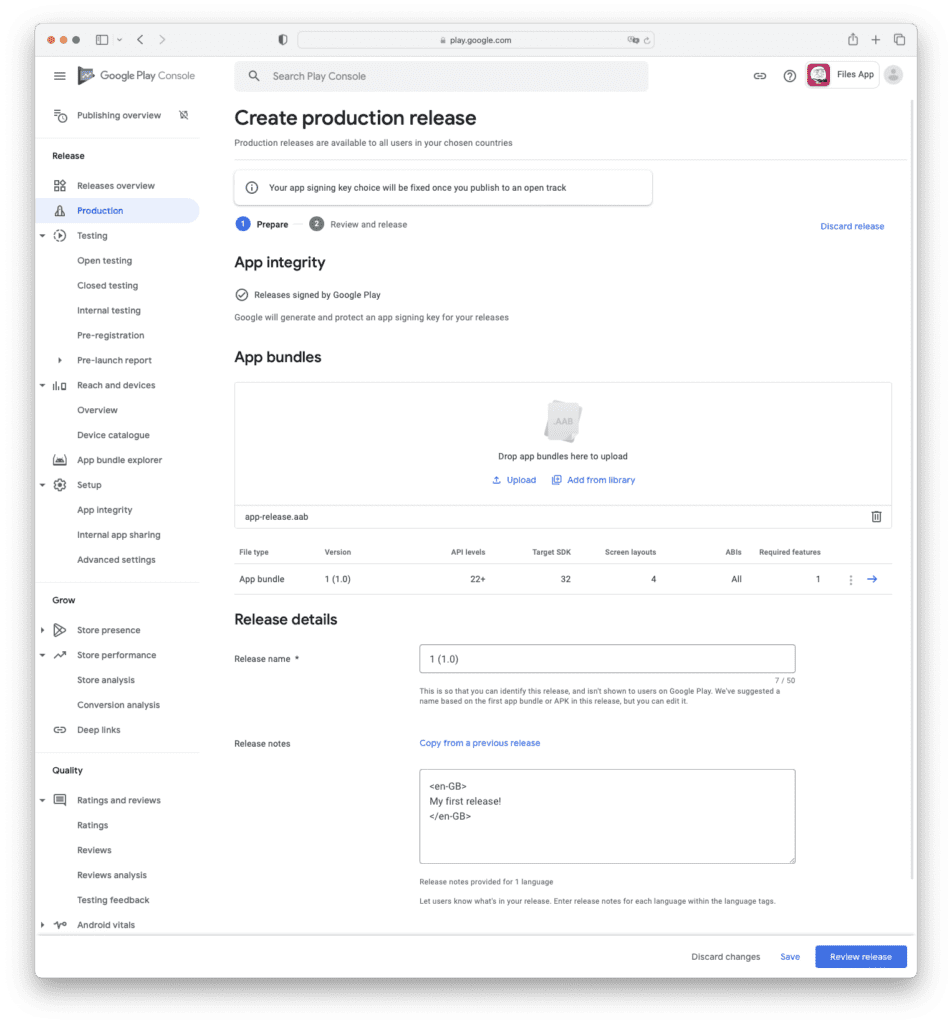
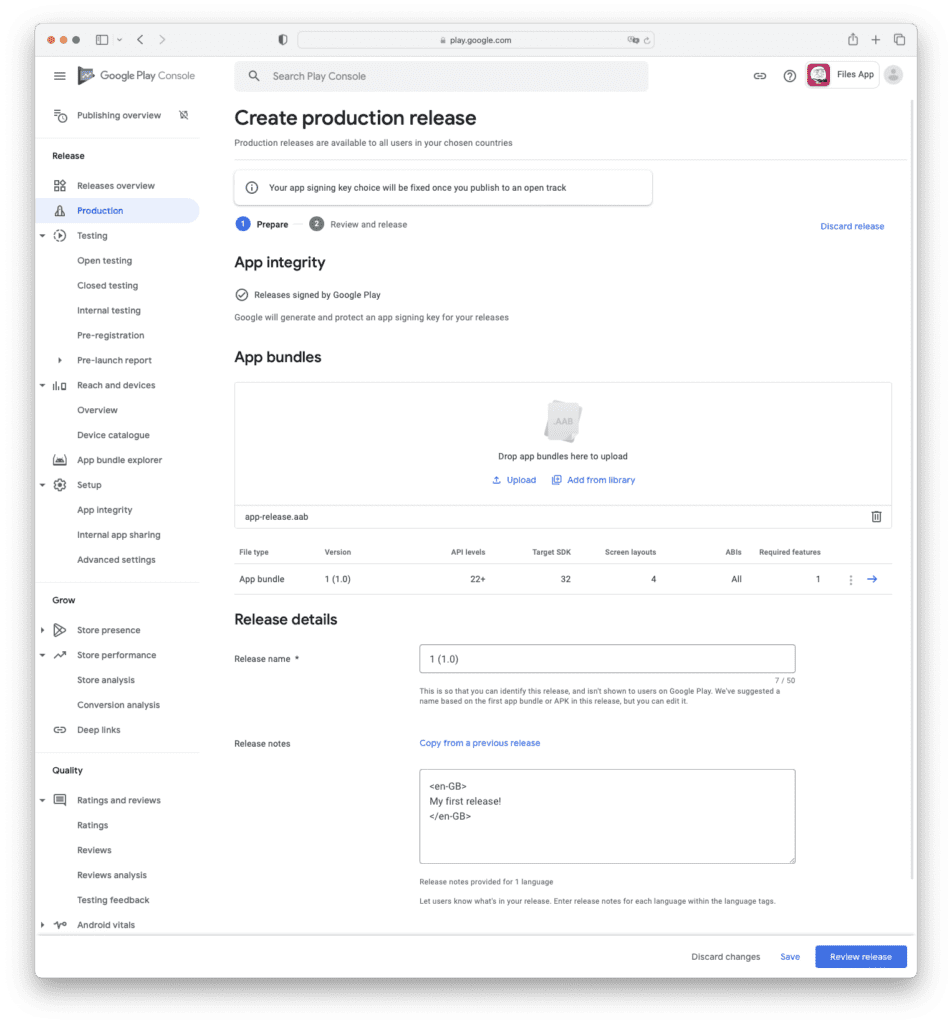
Google will automatically detect the version of our file, target SDK level, and other information.
If you did all the previous steps in the right order, the bundle should be accepted, and you can click on Review release.
At this point, you might see some warnings, but they are not blocking your release.
Before you click on Start roll-out to Production, keep in mind that this action will release your app to the Google Play Store (after they review it). If everything looks good to you, hit that button and wait for a review, which might take 1-5 days depending on how busy Google is!
Congratulations – after some waiting, your app will be available for everyone to download through the Play Store. 🎉
Updating Your App
Let’s jump forward in time a few weeks (honestly, sometimes it’s just a minute until the next release) when you want to update your app. This process is the same in many aspects, but a lot faster because you don’t have to fill out all the general information of your app again.
Android App Update Process
To update your Android app, you also need to generate a new signed bundle, but before you create the bundle, it’s time to update your app version.
For Android, this can be done inside the android/app/build.gradle of your Ionic project.
Within the defaultConfig block, you can increment the: – versionCode in order for the Play Store to recognize that there is a new version for users.
Sync your web assets with the Android project, save the new Gradle settings and then create a new signed bundle through Android Studio or the command line and upload it within your developer console as a new version!
Managing Settings with Trapeze
Although Capacitor will never magically overwrite your native projects, there is a handy package called Trapeze which allows applying changes to your projects based on a YAML file.
Especially if you run your builds on a server, Trapeze can automate the task of updating native project settings with a single command based on your configuration. You can also check out my video if you want to see Trapeze in action if you are curious and want to know more.
Live Updates with Appflow
Now wouldn’t it be nice if you didn’t have to worry about builds, signing, upload, or even app store review times anymore? Well, all of that is actually possible with Appflow, a paid cloud platform for teams building any kind of mobile applications – yes, Appflow can build Cordova, Capacitor, and even React Native and traditional iOS and Android apps!
Appflow can manage your signing keys, connect to your repository and automatically build your native iOS or Android app after pushing to a specific branch. By defining different pipelines, you can set up powerful automations, like automatically uploading a new build to Google or Apple after it’s been built in the cloud.
On top of that, you get access to a feature called live updates:
With live updates, you can actually skip the app store review process because apps connected with Appflow can load their web sources from a remote server.
There’s also an in-depth look at Appflow inside the Ionic Academy, so if you want to learn how to use it or build even better Ionic React apps, become a member and get immediate access to 70+ video courses, app templates and our private community of Ionic developers. ❤️
Parting Thoughts
While it can seem like a complex process, building, signing, and releasing your app is actually a lot more approachable than you might think. While this guide only covered Android, keep an eye out for the next guide which will go over this same process, but for iOS!