Ionic Native: Getting Your App Rated and Reviewed
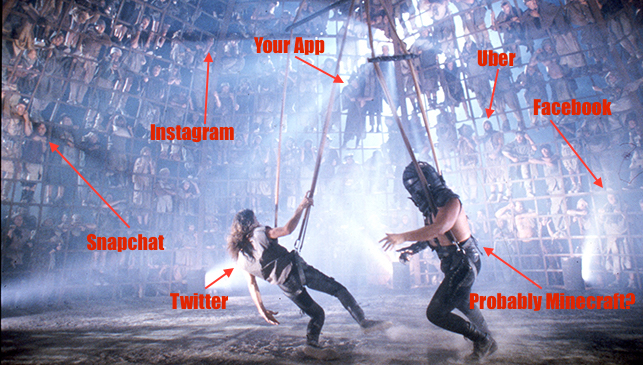
There’s lots of things that can make a mobile app successful–a great user experience, an intuitive and performant UI, not crashing, and actually being useful are just a few crazy ideas that just might work. But at the end of the day, apps first need to be discovered. There’s currently something to the tune of over two million apps in the Apple App Store and two million apps in the Google Play Store, all fighting for your attention in a dystopian, Thunderdome-like scenario. Conceptually it’s something like this:
What I’m trying to say is, there’s a lot of competition, which can make it very hard for a new app to get noticed, let alone downloaded. It’s pretty bleak. So how to triumph and rise to the top of the charts? One of the best ways to break out of the crowd is to get positive ratings and reviews from your users.
In this post, we’ll take a look at one of the most common ways to get users to rate and review apps: by asking them to. Specifically, we’ll take a look at the App Rate plugin in Ionic Native, which makes it easy to prompt users to rate and review your app.
It’s useful, simple, and best of all, it doesn’t require a 1980’s special effects hellscape to accomplish.
Why Should I Care About Ratings/Reviews?
Because they make you feel loved, and everyone wants to be loved, yes? Aside from that, here are just a few reasons why you might want positive ratings/reviews, and lots of them:
- Your app may appear higher in search results.
- If you make it to the top charts, your app is is more likely to stay there longer.
- Users are more likely to download your app AND try it.
The potential bottom line? More users, more downloads, more success, more $$$$. All important things if you’re an app developer.
Getting Set Up
To start, let’s add the Cordova App Rate plugin to our project with the Ionic CLI:
$ ionic plugin add cordova-plugin-apprate
Next, let’s get a little fancy. We might want the flexibility to be able to call the App Rate plugin to prompt the user from anywhere in our app, without having to configure it multiple times. A good way to do this is to configure a single instance of the plugin in a shared service that can be injected and called from any component in our app.
To create a new service, we can use the handy generate
command in the Ionic CLI to create a template for a new provider
:
$ ionic generate provider rate-service
This will create some boilerplate for us in /app/providers/rate-service/rate-service.ts
, where we will import the App Rate plugin. We’re also going to import Ionic’s Platform
plugin, which we’ll need a little later:
import { Injectable } from '@angular/core';
import { AppRate } from 'ionic-native';
import { Platform } from 'ionic-angular';
Next, we’ll also create an instance of AppRate
and Platform
in our constructor:
appRate: any = AppRate;
constructor(public platform: Platform) {
}
Configuring the Plugin
To make sure the user’s rating is applied to the correct app, we provide the App Rate plugin with our app’s package name for Android and App ID for iOS.
For iOS, this is the numeric App ID that is assigned to your app when you register it with iTunes Connect.
For Android, this is the id
attribute of the <widget>
tag in our project’s config.xml
file:
<widget id="com.ionicframework.apprate601311" version="0.0.1" ...>
When you create a new Ionic project using the Ionic CLI, this is set for you. By convention, reverse domain name format is used. You can (and should) feel free to change it to something custom before submitting your app to the App Store or Google Play Store. For iOS, this will act as the bundle identifier for your app.
Back to configuration.
We provide this to the App Rate plugin in our constructor. Notice that we need to wrap this in Ionic’s platform.ready()
. This ensures that Cordova has loaded the plugin before we begin working with it:
constructor(public platform: Platform) {
this.platform.ready().then(
() => this.appRate.preferences.storeAppURL = {
ios: '849930087',
android: 'market://details?id=com.ionic.viewapp'
}
)
}
To make things more straightforward, I’ve configured this example to point to the Ionic View app, since the example app we’re building isn’t actually available in the App Store or Google Play store.
At this point, there’s a variety of options we can set in the preferences
object of this.appRate
. Here’s a few of the more useful ones:
- usesUntilPrompt: Defers prompting the user until the
AppRate.promptForRating(false)
has been called a specified number of times. Note that this is persisted in local storage, so the user will be prompted again if they ever clear the app data or reinstall the app. - promptAgainForEachNewVersion: Prompts the user again if they have installed a newer version of the app.
- customLocale: A JSON object that specifies custom strings for use in the App Rate dialog. This can also be used for displaying different text for each language/locale. All of the following values must be set if you use this option:
- title: dialog title
- message: dialog body text
- cancelButtonLabel: text for cancel button
- laterButtonLabel: text for later button
- rateButtonLabel: text for rate button
So let’s go ahead and take advantage of some of these by changing our constructor to this:
constructor(public platform: Platform) {
this.platform.ready().then(
() => {
this.appRate.preferences= {
storeAppURL: {
ios: '849930087',
android: 'market://details?id=com.ionic.viewapp'
},
usesUntilPrompt: 2,
customLocale: {
title: 'Rate Us... Pretty Please?',
message: 'Without ratings we starve =(',
cancelButtonLabel: 'Pass',
rateButtonLabel: 'Rate it!',
laterButtonLabel: 'Ask Later'
}
}
}
)
}
For a full list of config options, see the Ionic Native App Rate docs.
Asking for a Review
Ok, let’s get those reviews flowing by injecting our shared service into a component of our app:
//import the service
import { RateService } from '../../providers/rate-service/rate-service';
//inject the provider for the service
@Component({
templateUrl: 'build/pages/home/home.html',
providers: [RateService]
})
export class HomePage {
//inject the shared service
constructor(public navCtrl: NavController, public rateService: RateService) {}
}
Now that we’re ready to roll, we have two options. We can ask for a rating any time by calling:
this.rateService.promptForRating();
Keep in mind that if we do this, the user will be asked to rate the app every time this code runs, every single time they use the app, and they will remember that we did that to them…which isn’t good. So it’s probably best not to do it, unless we’re careful about the application logic that determines when it’s called.
Option two is to let the config we set up handle when to trigger the prompt for us:
this.rateService.promptForRating(false);
Passing false
as an argument to the App Rate plugin tells it not to prompt the user until the conditions in our config have been met. In this case, since we set usesUntilPrompt: 2
, it won’t ask until promptForRating(false)
is called the second time, and only the second time.
So where should we call it? It’s advisable to ask the user to review or rate your app after they’ve done something that leaves them with a positive impression. Here’s a couple hypothetical examples of when it might be a good time to ask:
- In the constructor or
ionViewDidEnter()
of a confirmation page when the user has completed an order. - In the
onDidDismiss()
of a modal that delivered some valuable content to the user.
Basically, we want reviews and ratings, of course, but we also want to ask when the user is happy with the app. We also want to ask in a way that interrupts the experience as little as possible.
Trying it Out
In case you weren’t following along, I’ve made a sample app that provides solid value by letting you see three pictures of puppies before it prompts you for a review.
Now, all we have to do is test the app on a device with Ionic View with the App ID b0c526e8
:
Or by downloading it from GitHub and deploying to a device with ionic run <ios | android> --device
.
May your ratings always be high and your reviews plentiful.